make documents visible in NAV/BC
With the move to webclient-only Microsoft enables alot of new possibilities. Today we want to take a look on how we can make documents visible to the user without downloading them. Every browser is able to show documents like PDFs directly without any special libary. We can use this and just using a <iframe />
<iframe src="https://www.w3.org/WAI/ER/tests/xhtml/testfiles/resources/pdf/dummy.pdf"></iframe>
That simple! Since we got AL extension it is also easy to embbed HTML into NAV/BC.
Our extension just needs 3 different Files:
controladdin InlineFileControllerAddIn {
RequestedWidth = 600;
MinimumHeight = 600;
RequestedHeight = 600;
VerticalStretch = true;
HorizontalStretch = true;
Scripts =
'https://cdnjs.cloudflare.com/ajax/libs/jquery/3.2.1/jquery.js',
'AddIn/InlineFile/js/inlineFile.js';
StartupScript = 'AddIn/startup.js';
event OnControlAddInReady();
procedure CreateInlineFile();
}
$(document).ready(function () {
Microsoft.Dynamics.NAV.InvokeExtensibilityMethod('OnControlAddInReady', null);
});
function CreateInlineFile() {
$("#controlAddIn").empty();
$("#controlAddIn").append('<iframe id="FilePreview" src="https://www.w3.org/WAI/ER/tests/xhtml/testfiles/resources/pdf/dummy.pdf"></iframe>');
}
Now we can use this addin:
page 50000 Example {
// ...
usercontrol(InlineFileView;InlineFileAddIn)
{
ApplicationArea = All;
trigger OnControlAddInReady()
begin
CurrPage.InlineFileView.CreateInlineFile();
end;
}
// ...
}
And this is how it looks like:
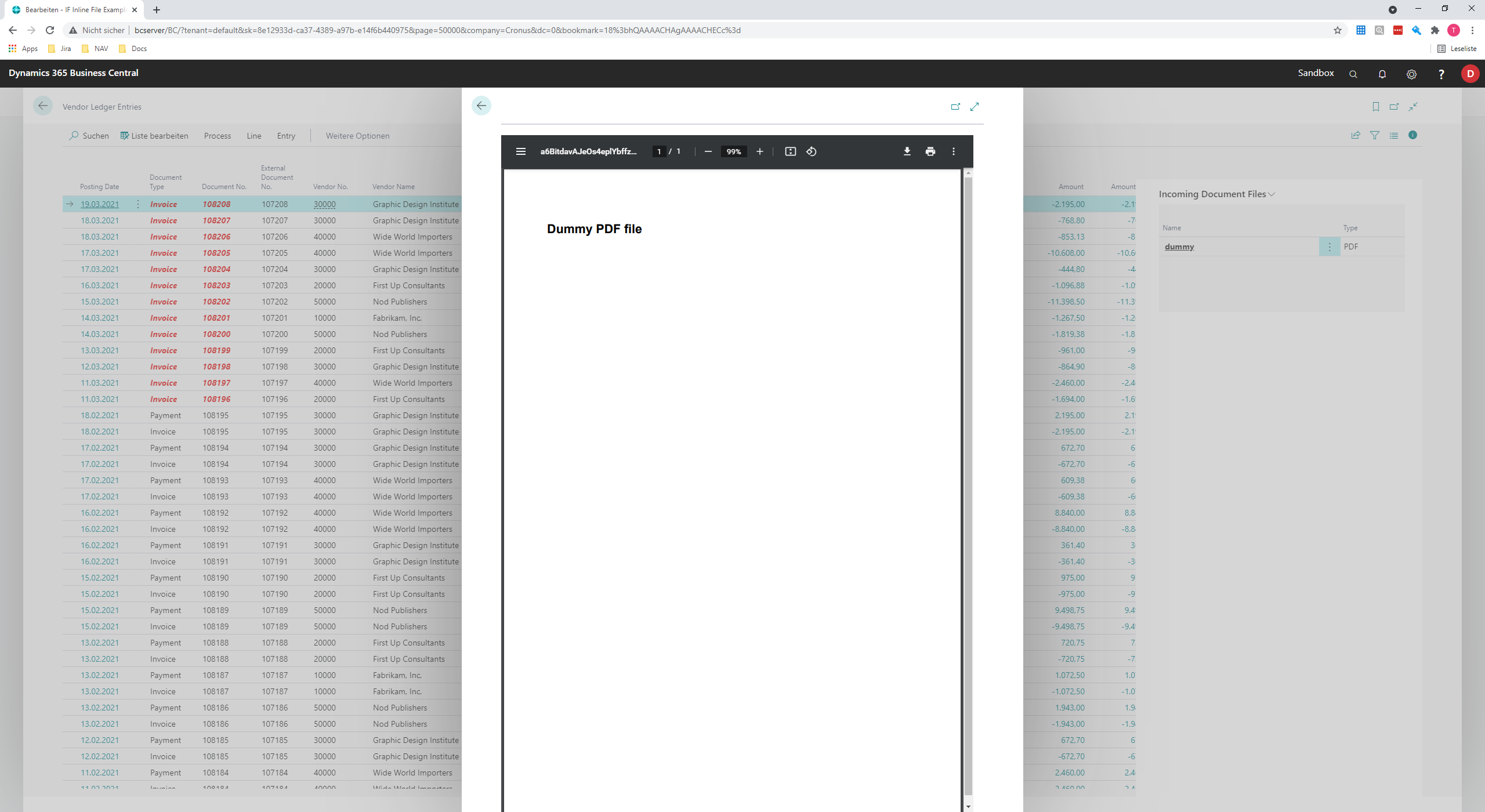
And thats it! We are able to show a PDF in NAV/BC without downloading it.
But wait. We can only show a static Document from somewhere. Okay okay lets adjust our function a bit:
function CreateInlineFile(Src) {
$("#controlAddIn").empty();
$("#controlAddIn").append('<iframe id="FilePreview"></iframe>');
$('#FilePreview').attr('src', Src);
}
Now we can pass different filelinks into the function. Its still not what you are looking for?
You are right, why do we need always a file somewhere? We dont! Browser are also able to show base64 content.
<iframe src="data:application/pdf;base64,YOUR_BINARY_DATA" height="100%" width="100%"></iframe>
Let us check another thing: Because of security reasons some companies block iframes – and this would mean, that this addin would not work. But instead of using a iframe we can also use <object>
. (read here)
Lets code this out:
controladdin InlineFileControllerAddIn {
RequestedWidth = 600;
MinimumHeight = 600;
RequestedHeight = 600;
VerticalStretch = true;
HorizontalStretch = true;
Scripts =
'https://cdnjs.cloudflare.com/ajax/libs/jquery/3.2.1/jquery.js',
'AddIn/InlineFile/js/inlineFile.js';
StartupScript = 'AddIn/startup.js';
event OnControlAddInReady();
procedure CreateInlineFile(Src: Text);
procedure SetSize(Height: Text; Width: Text);
}
var ObjectHeight = '100%', ObjectWith = '100%'
function SetSize(Height, Width) {
ObjectWith = Width
ObjectHeight = Height
}
function CreateInlineFile(Src) {
let style = 'width: ' + ObjectWith + '; height: ' + ObjectHeight +'; border:none;'
$("#controlAddIn").empty();
$("#controlAddIn").append('<object id="FilePreview" style="' + style +'"></object>');
$('#FilePreview').attr('data', Src);
}
and this is how we would use it now:
// ...
usercontrol(InlineFileView; InlineFileAddIn)
{
ApplicationArea = All;
trigger OnControlAddInReady()
var
TmpBlob: Codeunit "Temp Blob";
Base64Convert: Codeunit "Base64 Convert";
IStream: InStream;
begin
TmpBlob.FromRecord(Rec, 8);
TmpBlob.CreateInStream(IStream);
CurrPage.InlineFileView.CreateInlineFile(StrSubstNo('data:%1;base64,%2', GetContentType(), Base64Convert.ToBase64(IStream)));
end;
}
>> complete code here <<
Addin also works (with view codechanges) in NAV 2018 Webclient. Windows Client is not supported
Try it out!
2 Comments